Task Description
Your task is to create a JavaScript function that injects stars into the DOM and add an event listener to a button that calls the function when clicked.
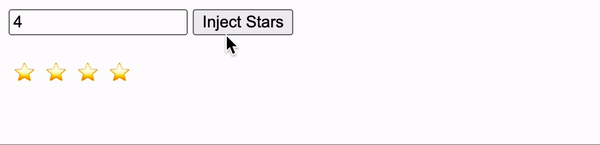
Requirements
- Create a function called
injectStars
that takes a number as an argument. - The function should remove all stars from the DOM before injecting new ones.
- The function should inject the number of stars passed in using a
for
loop andsetTimeout
to inject the stars one at a time. - Each star should be a
div
element with the classes "star" and "animate-star" and the innerHTML set to a star emoji ("⭐"). - The stars should be appended to the
body
of the document. - Create an event listener for a button element that calls the
injectStars
function with the number of stars to inject when clicked. - The number of stars to inject should be obtained from an input element.
Expected Output
When the button is clicked, the function should inject the specified number of stars into the DOM one at a time with a delay between each injection. The stars should be animated and should create a nice visual effect.
Example Code
Here's an example of what the code might look like:
function injectStars(num) {
// remove all stars from the DOM
const stars = document.querySelectorAll(".star");
stars.forEach((star) => star.remove());
// inject the number of stars passed in
// settimeout to inject the stars one at a time
for (let i = 0; i < num; i++) {
setTimeout(() => {
const star = document.createElement("div");
// add the star class and animate-star class
star.classList.add("star");
star.classList.add("animate-star");
star.innerHTML = "⭐";
document.body.appendChild(star);
}, i * 100);
}
}
// create event listener for the button
const button = document.querySelector("button");
// onclick, call the function and pass in the number of stars to inject
button.addEventListener("click", () => {
const input = document.querySelector("input");
injectStars(input.value);
});
This code creates a function called injectStars
that removes all stars from the DOM and injects new ones using a for
loop and setTimeout
. It also creates an event listener for a button element that calls the injectStars
function with the number of stars to inject when clicked.
Detailed Explanation
The first function we encounter is document.querySelectorAll
. This function returns a list of all elements in the document that match a specified CSS selector. In this case, the selector is ".star", which matches all elements with the class "star". The function is used to remove all stars from the DOM before injecting new ones.
The next function we see is forEach
. This is a method of arrays that calls a provided function once for each element in the array. In this case, the function is an arrow function that calls the remove
method on each star element.
The setTimeout
function is used to inject the stars one at a time with a delay between each injection. This creates a nice animation effect. The function takes two arguments: a function to execute and a delay in milliseconds. In this case, the function is an arrow function that creates a new div
element and adds the classes "star" and "animate-star" to it. It then sets the innerHTML
of the element to a star emoji ("⭐") and appends the element to the body
of the document.
To use this function, you can create an event listener for a button or other element and call the function when the event is triggered. For example:
const button = document.querySelector("button");
button.addEventListener("click", () => {
const input = document.querySelector("input");
const numStars = parseInt(input.value);
injectStars(numStars);
});
In this example, we select a button element and add an event listener for the "click" event. When the button is clicked, we select an input element and get its value as a number. We then call the injectStars
function with the number of stars to inject.
And that's it! With just a few lines of JavaScript, you can inject stars into the DOM.
Hope you learned something out of this. Happy Coding.